Anchor (Beta)
In virtual reality (VR), an anchor is used to maintain a consistent correspondence between virtual objects and specific locations in the real world, even when there is a reset of the headset's pose (HMD). This feature ensures that, regardless of changes in the user's viewpoint or orientation caused by resetting the headset, the virtual objects remain accurately positioned in relation to the real-world environment. Anchors effectively link virtual content to a fixed point in physical space, allowing for a coherent and consistent user experience, where virtual elements reliably appear in the same real-world locations, despite any adjustments in the headset's tracking or calibration.
Supported Platforms and Devices
Platform | Headset | Supported | Plugin Version | |
PC | PC Streaming | Focus 3/XR Elite/Focus Vision | X | |
Pure PC | Vive Cosmos | X | ||
Vive Pro series | X | |||
AIO | Focus 3/XR Elite/Focus Vision | V | 2.3.0 and above |
Specification
This chapter dives into creating immersive experiences with the Anchor feature. We'll explore its use within the Anchor extension.
Environment Settings
The feature depend on HTC XR Elite v1.0.999.644 or newer ROM.
VIVE OpenXR Unity plugin supports Anchor VIVE XR Anchor which depends on the OpenXR feature group.
Enable the Anchor feature in Edit > Project Settings > XR Plug-in Management > OpenXR, enable the VIVE XR Anchor feature.
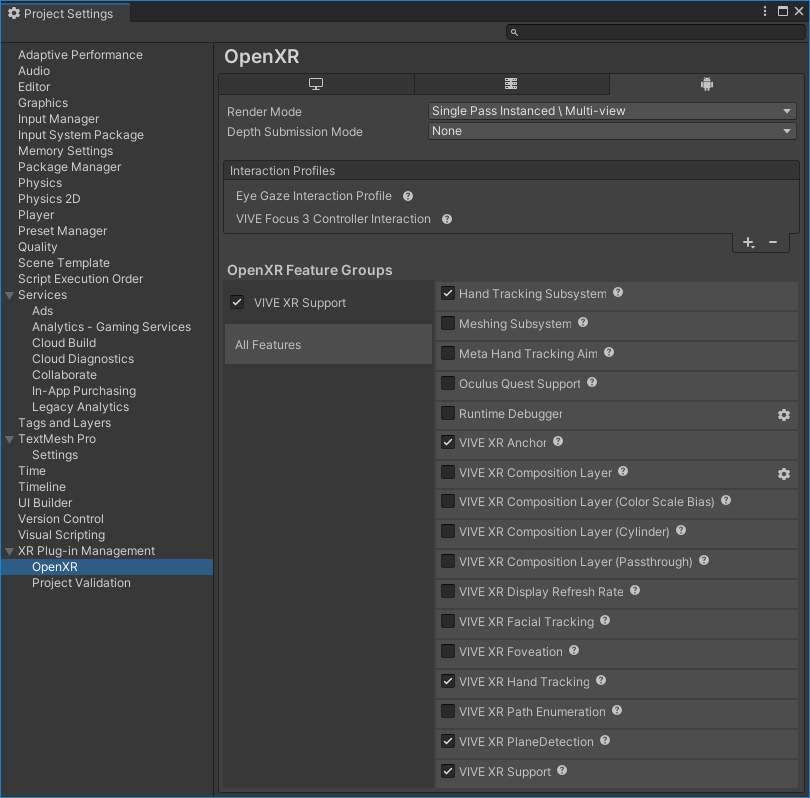
Golden Sample
The Sample at Asset > VIVE > OpenXR > > Samples > Anchor.
How to use Anchor
A spatial anchor is mapping to real-world item. You can track the real-world item by the anchor. In practice, we create an anchor with passthrough image opened. When the Passthrough is enabled, you can see the real-world item, and use controller to get real-world item’s pose in VR’s tracking space to map real-world pose with virtual-world pose.
You can refer to the script AnchorTestHandle.cs.
1. Check if the Anchor feature is supported.
if (!AnchorManager.IsSupported()) return;
2. Create an Anchor.
To create an Anchor, which requires the Pose relative to the TrackingOrigin and a unique name. Here, the frameCount is used in the name to avoid duplication with others.
AnchorManager.Anchor anchor = AnchorManager.CreateAnchor(relatedPose, name + " (" + Time.frameCount + ")");
3. Get the Pose of the Anchor.
AnchorManager.GetTrackingSpacePose(anchor, out Pose pose);
4. Get the name of the Anchor.
anchor.GetSpatialAnchorName();
See Also
Anchor extension.
FAQ
The base anchor does not persist after the application is restarted. To retain the anchor, a persistent anchor must be utilized. The development of the persistent extension is currently ongoing.